Maybe that could be of help, I’ve been fiddling with the JS API and made myself a tool to search through code because Squiz will take way to long to implement that.
DESIGN Parse file:
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html lang="en">
<!-- ASSET ID: <MySource_PRINT id_name="__global__" var="assetid" /> -->
<head>
<title><MySource_PRINT id_name="__global__" var="site_name" />: <MySource_PRINT id_name="__global__" var="asset_name" /></title>
<!-- META DATA -->
<meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1">
<mysource_area id_name="metadata" design_area="metadata" />
<!-- BOOTSTRAP -->
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0-beta/css/bootstrap.min.css" integrity="sha384-/Y6pD6FV/Vv2HJnA6t+vslU6fwYXjCFtcEpHbNJ0lyAFsXTsjBbfaDjzALeQsN6M" crossorigin="anonymous">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.11.0/umd/popper.min.js" integrity="sha384-b/U6ypiBEHpOf/4+1nzFpr53nxSS+GLCkfwBdFNTxtclqqenISfwAzpKaMNFNmj4" crossorigin="anonymous"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0-beta/js/bootstrap.min.js" integrity="sha384-h0AbiXch4ZDo7tp9hKZ4TsHbi047NrKGLO3SEJAg45jXxnGIfYzk4Si90RDIqNm1" crossorigin="anonymous"></script>
<!-- SQUIZ MATRIX JS API -->
<script type="text/javascript" src="./?a=387261"></script>
</head>
<body>
<MySource_AREA id_name="body" design_area="body" />
</body>
</html>
HTML:
<div class="container">
<div class="row">
<div class="col-sm-3">
</div>
<div class="col-sm-6">
<h1>Serge's Search Tool</h1>
<form onsubmit="onSubmit()">
<div class="form-group">
<label for="node">Parent Asset:</label>
<input type="numeric" class="form-control" id="node" value="28673">
</div>
<div class="form-group">
<label for="search">Search:</label>
<input type="search" class="form-control" id="search" value="class">
</div>
<button type="submit" class="btn btn-default">Submit</button>
</form>
</div>
<div class="col-sm-3">
</div>
</div>
<div class="row">
<div id='log' class="col-sm-12"></div>
</div>
</div>
<iframe name="iframe1" src="target.html" height="100%" width="100%"></iframe>
Javascript:
<script>
function add_result(id, type) {
var msg_class = "";
switch(type) {
case "css_file": msg_class="badge-primary"; break;
case "js": msg_class="badge-secondary"; break;
case "html": msg_class="badge-success"; break;
case "wysiwyg": msg_class="badge-warning"; break;;
case "design": msg_class="badge-danger"; break;
default: console.log("Unknown type: ", type);
}
// <span class="label label-success">Success</span>
var url = "/_admin_sandpit/?assetid="+id+"&am_section=edit_asset";
$('#log').append("<a href='" + url +"' class='badge " + msg_class + "' target='iframe1'>" + id + "</a> \n");
}
function search_css(search, css_asset) {
$.ajax({ url: css_asset.web_path,
dataType: 'javascript',
success: function(data) {
search_in_string(css_asset.asset_id, "css", search, data);
}
});
}
function search_js(search, js_asset) {
console.log(js_asset.asset_id, "/js");
$.ajax({ url: js_asset.web_path,
dataType: 'javascript',
success: function(data) {
search_in_string(js_asset.asset_id, "js", search, data);
}
});
}
function search_html(search,html_asset) {
if (html_asset.type_code != 'content_type_raw_html') {
console.log("Seems like the asset you've given me isn't content_type_raw_html: ", html_asset);
return null;
}
search_in_string(html_asset.asset_id, "js", search, html_asset.html);
}
function search_wysiwyg(search, wysiwyg_asset) {
// NOTE: This is virtually the same as an html asset
if (wysiwyg_asset.type_code != 'content_type_wysiwyg') {
console.log("Seems like the asset you've given me isn't content_type_wysiwyg: ", wysiwyg_asset);
return null;
}
search_in_string(wysiwyg_asset.asset_id, "wysiwyg", search, wysiwyg_asset.html);
}
function search_design(search, design_asset) {
// Make sure it's a design asset
// Iterate through the contents array, replace in _type = "HTML"
// {_type: "HTML", contents: "<html><tags> ..."}
var id = design_asset.asset_id
var original_contents = design_asset.contents;
var updated_contents = [];
var found_list = [];
var line = {};
var n = -1;
var found = 0;
// contents = [{_type: "HTML", contents: "<html>code line..."}, ...]
for(var i=0; i < original_contents.length;i++) {
line = original_contents[i];
// Replace content of HTML lines ONLY
if(line._type == "HTML") {
search_in_string(design_asset.asset_id, "design", search, line.contents);
}
}
}
// THESE ARE WORKING ////////////////////////////////////////////
function load_asset_list() {
var asset_types_to_update = ["css_file", "js_file", "content_type_raw_html","content_type_wysiwyg","design"];
console.log("Loading Assets to update ", asset_types_to_update,"...");
matrix_js_api.getChildren({
"asset_id": main_node,
"levels":20,
"type_codes":asset_types_to_update,
"get_attributes":1,
"dataCallback": asset_list_loaded
})
}
function search_in_assets(asset_list) {
if(!Array.isArray(asset_list)) {
console.log("Something wrong with this asset list, expecting an array ... ", asset_list);
alert("Problem with asset list, check console.");
return null;
}
console.log("Iterating through asset_list ... ", asset_list);
for(var i=0; i < asset_list.length;i++) {
search_asset(asset_list[i]);
}
}
function asset_list_loaded(asset_list) {
if(typeof asset_list === 'Array') {
console.log("Something wrong with this asset list, expecting an array ... ", asset_list);
alert("Problem with JSON response, check console.");
}
search_in_assets(asset_list);
}
function search_asset(asset) {
if (typeof asset.type_code !== 'string') {
console.log("Seems like the asset you've given me doesn't have a type_code: ", asset);
return null;
}
switch(asset.type_code) {
case "css_file": search_css(search, asset); break;
case "js_file": search_js(search, asset); break;
case "content_type_raw_html": search_html(search, asset); break;
case "content_type_wysiwyg": search_wysiwyg(search, asset); break;
case "design": search_design(search, asset); break;
default:
console.log("Fatal error, asset type: ", asset.type_code, " is not handled.");
}
return null;
}
function search_in_string(id, type, needle, haystack) {
// Look for a string and return it with its context for logging purpose
var n = haystack.search(needle);
if(n > -1) {
// Returns a substring with context, removing the carriage returns
var sample = haystack.substring(n, n + search_lenght);
add_result(id, type);
} else {
// tell_user("Asset: <a href=''>" + id + "</a> (" + type + ") --> Nothing found", "info");
}
}
function onSubmit() {
event.preventDefault(); // Stop the page from loading a new URL
search = new RegExp($("#search").val(), 'g');
search_lenght = $("#search").val().length;
main_node = $("#node").val();
$("#log").empty();
//alert("You are searching for: " + search);
// Load all asset content in a matrix.
load_asset_list();
// Replace all content and put in new matrix.
// updated_asset_list = replace_in_matrix(search, replace, original_asset_list);
// Update all assets that have been changed.
//update_asset_list(updated_asset_list);
}
// CACHE HANDLING ////////////////////////////////////////////
//
//lastrun = localStorage.getItem('lastrun');
//if(lastrun == null) {
// self.load_users();
//} else {
// self.data = JSON.parse(localStorage.getItem('mydata'));
// $('#mylist').bootstrapTable({
// data: self.data
// });
//}
// GLOBALS ////////////////////////////////////////////////////
//
var search = "";
var replace = "";
var search_length = 0;
var main_node = 1; // Use 1 for Root
var options = new Array();
options['key'] = '6970512344';
matrix_js_api = new Squiz_Matrix_API(options);
</script>
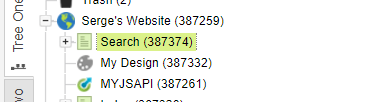
Note that this is a working draft, I haven’t had time to spend on this tool the moment it was working I was able to finish the job I had to do. If there is a need for better tools across the community I could spend more time on it, as long as Squiz fixes their JS API so we can actually properly manipulate the backend.